Code Formatter
A code formatter plugin adds support for a new language or formatting scheme. A menu item is added to the Edit / Code Formatting
menu and the key binding will trigger your plugin.
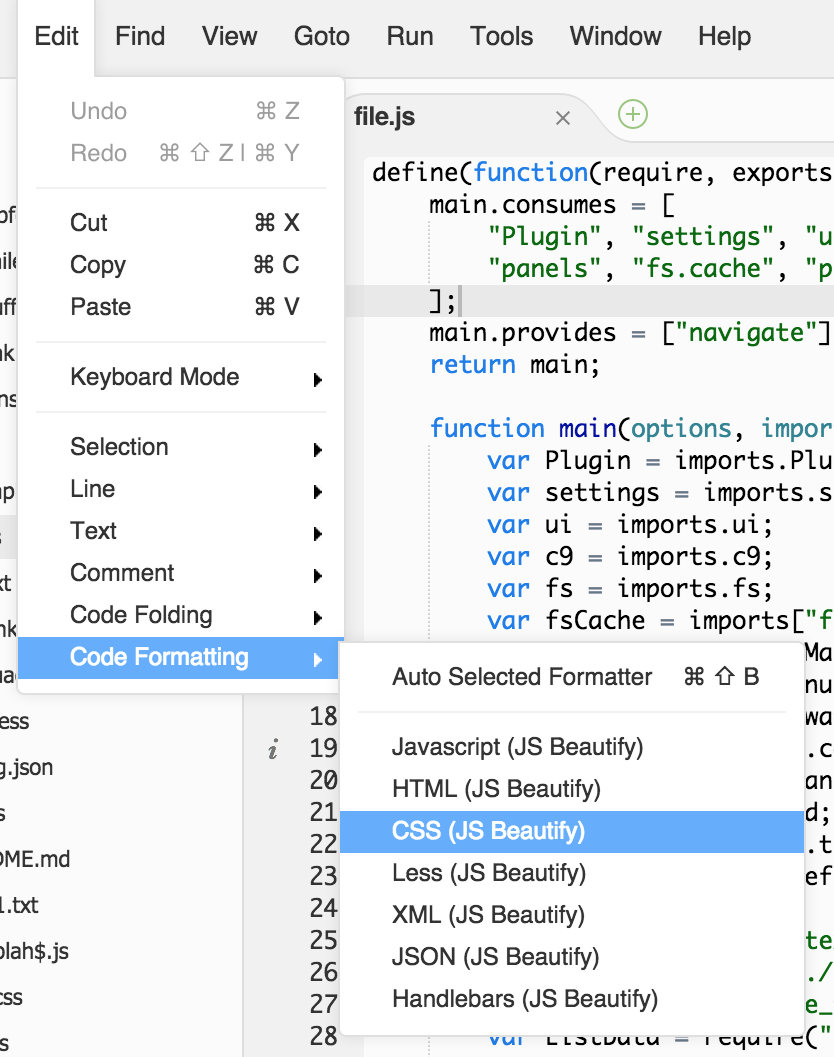
Adding the Menu Item
In the load
event of your plugin call addFormatter()
to add the menu item to the Edit menu.
format.addFormatter("Javascript (JS Beautify)", "javascript", plugin);
Responding to a Format Request
Listen to the format
event on the format plugin. This event is called for each format request. Your plugin can decide whether to respond to the event or not. Make sure to return true
after executing the formatting.
format.on("format", function(e) {
if (e.mode == "javascript")
return formatCode(e.editor, e.mode);
});
Format Ace's Selection
The format function gets a reference to the editor object and the mode. The following example retrieves the value of the selection, replaces the value and selects the new content again.
function formatCode(editor, mode) {
var ace = editor.ace;
var sel = ace.selection;
var session = ace.session;
var range = sel.getRange();
var value = session.getTextRange(range);
value = doFormatting(value);
var end = session.diffAndReplace(range, value);
sel.setSelectionRange(Range.fromPoints(range.start, end));
return true;
}
Full Example
See the jsbeautify implementation for a full example.
Updated about 2 months ago